How Developers Can Make improvements to Their Debugging Techniques By Gustavo Woltmann
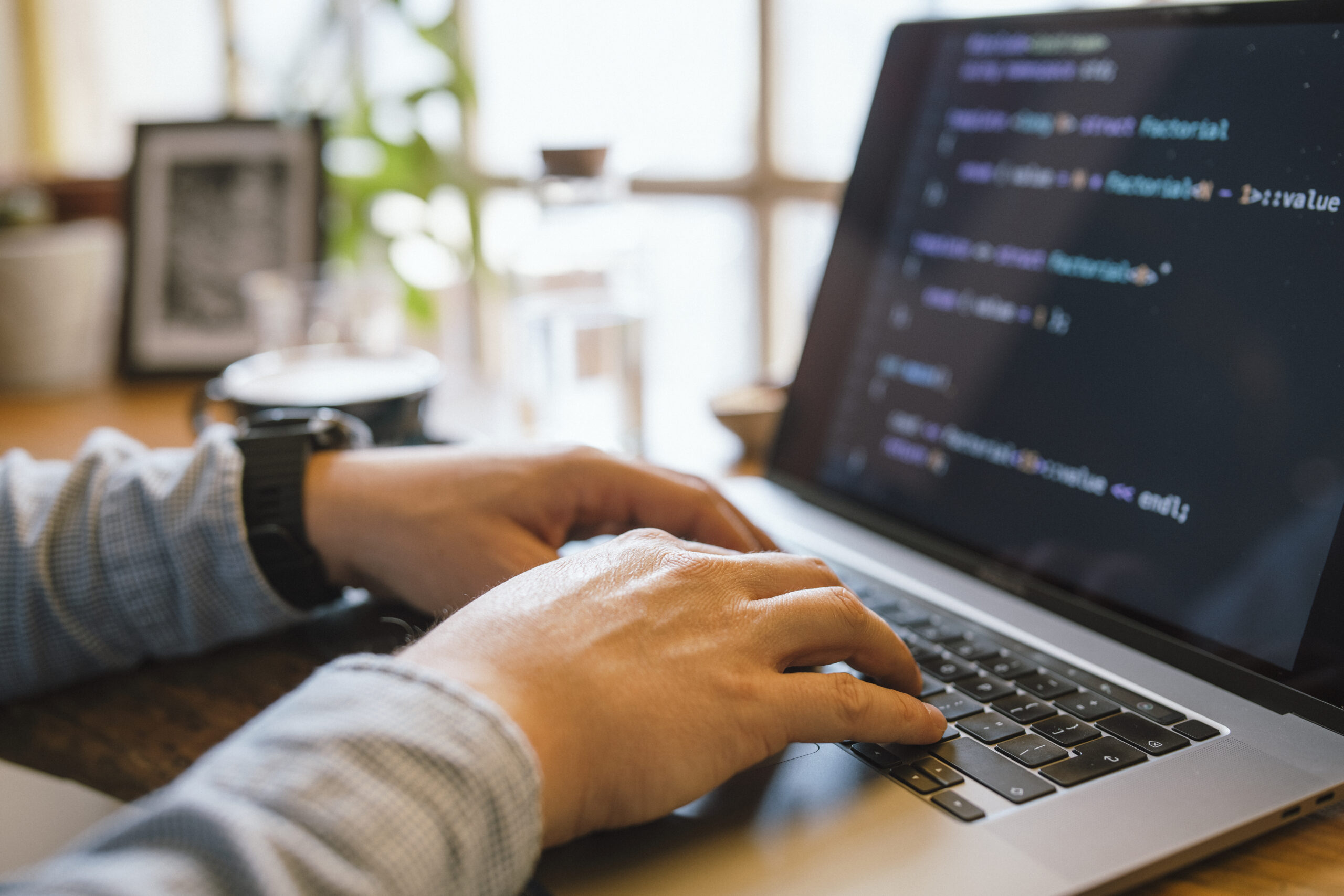
Debugging is The most critical — however normally overlooked — expertise in a developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why factors go Incorrect, and Studying to Feel methodically to resolve difficulties proficiently. No matter whether you are a starter or simply a seasoned developer, sharpening your debugging competencies can help you save several hours of annoyance and dramatically enhance your productivity. Here are a number of methods to help builders level up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest methods developers can elevate their debugging abilities is by mastering the applications they use everyday. When composing code is a single Section of improvement, knowing ways to communicate with it efficiently throughout execution is equally important. Modern-day growth environments arrive equipped with highly effective debugging capabilities — but several developers only scratch the floor of what these resources can perform.
Get, for example, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources allow you to set breakpoints, inspect the value of variables at runtime, move by means of code line by line, and even modify code about the fly. When utilized effectively, they Allow you to observe just how your code behaves during execution, that's invaluable for monitoring down elusive bugs.
Browser developer tools, such as Chrome DevTools, are indispensable for front-close developers. They help you inspect the DOM, keep track of community requests, perspective authentic-time efficiency metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can convert frustrating UI challenges into manageable duties.
For backend or process-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB give deep Regulate over working procedures and memory management. Understanding these instruments can have a steeper Finding out curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into snug with version Management units like Git to understand code background, uncover the precise minute bugs ended up introduced, and isolate problematic adjustments.
Eventually, mastering your instruments suggests likely further than default configurations and shortcuts — it’s about building an intimate understanding of your development atmosphere to ensure when troubles occur, you’re not shed at the hours of darkness. The greater you know your tools, the more time you could expend resolving the particular challenge in lieu of fumbling by the process.
Reproduce the Problem
Probably the most critical — and often missed — ways in helpful debugging is reproducing the problem. Before leaping in the code or generating guesses, developers need to produce a consistent ecosystem or state of affairs the place the bug reliably appears. With out reproducibility, correcting a bug will become a video game of possibility, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a dilemma is accumulating just as much context as you possibly can. Talk to issues like: What actions triggered The problem? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater element you might have, the simpler it results in being to isolate the precise situations under which the bug happens.
When you’ve gathered sufficient information and facts, seek to recreate the trouble in your neighborhood surroundings. This may suggest inputting the same knowledge, simulating equivalent person interactions, or mimicking program states. If The difficulty appears intermittently, take into account writing automated tests that replicate the edge conditions or state transitions involved. These exams don't just assist expose the situation but in addition prevent regressions Later on.
From time to time, the issue could be ecosystem-particular — it'd occur only on specified functioning systems, browsers, or below unique configurations. Applying equipment like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these kinds of bugs.
Reproducing the condition isn’t just a stage — it’s a mentality. It requires persistence, observation, as well as a methodical approach. But when you can persistently recreate the bug, you happen to be by now halfway to fixing it. Using a reproducible situation, You need to use your debugging instruments additional correctly, check prospective fixes securely, and talk far more Plainly using your crew or end users. It turns an summary criticism right into a concrete problem — and that’s in which developers thrive.
Read and Recognize the Error Messages
Error messages will often be the most precious clues a developer has when a little something goes Completely wrong. Rather then viewing them as irritating interruptions, developers ought to learn to take care of mistake messages as direct communications from the method. They often show you just what exactly occurred, exactly where it transpired, and from time to time even why it occurred — if you understand how to interpret them.
Begin by reading the concept very carefully As well as in whole. Several builders, specially when beneath time force, glance at the main line and promptly begin earning assumptions. But further inside the error stack or logs may possibly lie the accurate root induce. Don’t just copy and paste mistake messages into engines like google — study and comprehend them to start with.
Break the mistake down into components. Could it be a syntax error, a runtime exception, or possibly a logic mistake? Will it stage to a certain file and line quantity? What module or purpose triggered it? These inquiries can guide your investigation and position you toward the dependable code.
It’s also useful to comprehend the terminology of your programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java normally stick to predictable styles, and Finding out to acknowledge these can significantly accelerate your debugging system.
Some mistakes are obscure or generic, and in All those situations, it’s very important to examine the context during which the mistake happened. Check connected log entries, enter values, and recent adjustments from the codebase.
Don’t overlook compiler or linter warnings either. These usually precede larger sized issues and provide hints about prospective bugs.
Ultimately, error messages are usually not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint difficulties a lot quicker, cut down debugging time, and become a far more successful and self-assured developer.
Use Logging Sensibly
Logging is one of the most powerful resources inside a developer’s debugging toolkit. When employed correctly, it offers serious-time insights into how an software behaves, serving to you have an understanding of what’s going on underneath the hood while not having to pause execution or action through the code line by line.
A good logging method begins with realizing what to log and at what degree. Typical logging ranges include DEBUG, INFO, WARN, Mistake, and FATAL. Use DEBUG for detailed diagnostic information all through enhancement, Details for standard activities (like prosperous start off-ups), WARN for possible challenges that don’t crack the appliance, ERROR for precise challenges, and Lethal once the method can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant information. Too much logging can obscure significant messages and slow down your technique. Concentrate on key gatherings, condition changes, enter/output values, and critical conclusion factors within your code.
Format your log messages Obviously and consistently. Include things like context, including timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting This system. They’re Primarily useful in output environments in which stepping as a result of code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, intelligent logging is about stability and clarity. That has a nicely-imagined-out logging solution, you are able to decrease the time it's going to take to spot difficulties, acquire further visibility into your applications, and improve the Total maintainability and here trustworthiness of your code.
Feel Just like a Detective
Debugging is not merely a specialized process—it is a method of investigation. To successfully recognize and deal with bugs, builders must method the method similar to a detective solving a mystery. This state of mind aids stop working advanced challenges into workable parts and adhere to clues logically to uncover the basis bring about.
Get started by accumulating proof. Think about the indications of the problem: error messages, incorrect output, or overall performance problems. The same as a detective surveys against the law scene, collect just as much applicable information as you can without leaping to conclusions. Use logs, exam conditions, and user reports to piece together a transparent photo of what’s occurring.
Following, kind hypotheses. Request your self: What might be causing this conduct? Have any modifications recently been made into the codebase? Has this challenge transpired just before under identical situation? The intention will be to slim down choices and identify opportunity culprits.
Then, take a look at your theories systematically. Make an effort to recreate the issue inside of a managed atmosphere. When you suspect a particular function or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, ask your code issues and Allow the effects guide you closer to the reality.
Pay out close consideration to little aspects. Bugs typically hide while in the least envisioned areas—similar to a missing semicolon, an off-by-a person error, or simply a race problem. Be complete and individual, resisting the urge to patch The difficulty without having fully knowledge it. Short-term fixes may well hide the true trouble, only for it to resurface later on.
Last of all, preserve notes on That which you tried and discovered. Just as detectives log their investigations, documenting your debugging system can conserve time for foreseeable future issues and aid Some others comprehend your reasoning.
By contemplating similar to a detective, builders can sharpen their analytical techniques, approach difficulties methodically, and turn into more practical at uncovering concealed problems in intricate devices.
Write Tests
Creating assessments is one of the most effective approaches to increase your debugging competencies and overall improvement effectiveness. Exams not merely support capture bugs early but also serve as a safety net that gives you self-assurance when generating improvements on your codebase. A perfectly-analyzed software is much easier to debug since it permits you to pinpoint just the place and when a challenge happens.
Begin with unit exams, which concentrate on person functions or modules. These small, isolated exams can rapidly reveal whether or not a specific bit of logic is Performing as predicted. Each time a examination fails, you right away know in which to appear, significantly lowering the time used debugging. Device exams are Particularly helpful for catching regression bugs—issues that reappear just after Earlier getting set.
Future, combine integration assessments and stop-to-finish checks into your workflow. These enable make certain that numerous aspects of your software function alongside one another efficiently. They’re especially handy for catching bugs that take place in complex devices with several components or expert services interacting. If one thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Producing tests also forces you to definitely think critically regarding your code. To test a attribute correctly, you require to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of knowing Normally sales opportunities to better code construction and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the take a look at fails consistently, it is possible to concentrate on repairing the bug and check out your test move when The difficulty is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from the irritating guessing match right into a structured and predictable system—assisting you catch far more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult situation, it’s uncomplicated to be immersed in the problem—watching your display screen for hrs, seeking Alternative after Answer. But One of the more underrated debugging tools is simply stepping away. Taking breaks helps you reset your mind, decrease annoyance, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for also extended, cognitive tiredness sets in. You could commence overlooking apparent mistakes or misreading code that you just wrote just hrs previously. On this state, your Mind results in being fewer successful at challenge-fixing. A short wander, a espresso split, or maybe switching to a unique endeavor for ten–15 minutes can refresh your concentrate. Many builders report obtaining the basis of a problem when they've taken time and energy to disconnect, allowing their subconscious function during the qualifications.
Breaks also aid avert burnout, Specifically throughout for a longer period debugging periods. Sitting before a display, mentally stuck, is not simply unproductive but additionally draining. Stepping absent lets you return with renewed Vitality and a clearer way of thinking. You could abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you in advance of.
Should you’re trapped, a superb rule of thumb is usually to set a timer—debug actively for 45–sixty minutes, then take a five–10 minute break. Use that point to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily beneath limited deadlines, however it essentially results in speedier and more effective debugging Ultimately.
In brief, taking breaks is just not an indication of weakness—it’s a wise tactic. It gives your brain Place to breathe, increases your viewpoint, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is usually a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you come across is much more than simply A short lived setback—It is a chance to improve to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, every one can educate you a thing important if you take some time to mirror and examine what went Erroneous.
Get started by inquiring yourself a couple of important queries after the bug is settled: What induced it? Why did it go unnoticed? Could it are caught before with better practices like unit tests, code assessments, or logging? The responses normally expose blind places as part of your workflow or knowledge and assist you Make much better coding behaviors transferring forward.
Documenting bugs can even be an outstanding habit. Keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. Over time, you’ll begin to see designs—recurring concerns or frequent errors—that you can proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with all your friends might be Specifically potent. Whether it’s via a Slack concept, a brief produce-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar concern boosts team effectiveness and cultivates a stronger Discovering tradition.
A lot more importantly, viewing bugs as classes shifts your frame of mind from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as essential areas of your development journey. In spite of everything, a number of the most effective developers are certainly not the ones who produce ideal code, but people that constantly study from their errors.
In the long run, Each and every bug you take care of adds a different layer to your ability established. So next time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Improving your debugging capabilities usually takes time, follow, and tolerance — however the payoff is big. It tends to make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, remember: debugging isn’t a chore — it’s a chance to become superior at Anything you do.